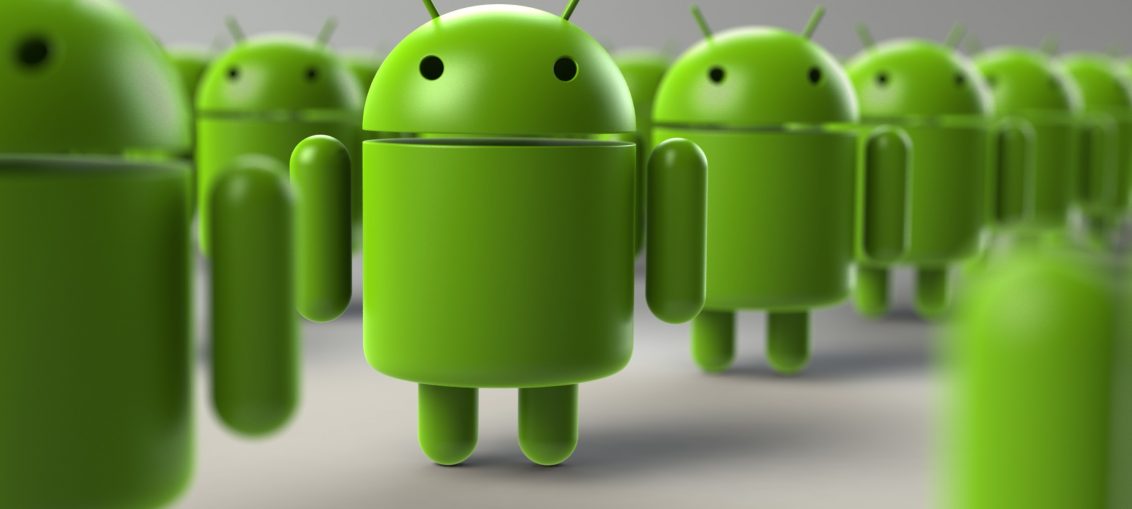
Kali ini kita akan melanjutkan android socket client dengan menambahkan activity age calculation. Activity atau view ini akan digunakan untuk menangani fungsi age calculation yang bisa dihandle oleh server socket update.
Selain meminta server untuk menghitung umur, android client ini juga dapat melakukan perhitungan umur sendiri. Karena ada fungsi menghitung umur di activity ini.
Langsung saja, kita create Blank Activity lalu kita beri nama AgePlanetActivity. Lalu akan terbentuk 2 file (java dan xml).
File “AgePlanetActivity.java”
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 |
public class AgePlanetActivity extends ActionBarActivity { private Socket socket; private static final int SERVERPORT = 6789; private static final String SERVER_IP = "10.0.2.2"; private String[] arrayPlanet; private String selectPlanet; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_age_planet); this.arrayPlanet = new String[] {"All","Mercury","Venus","Jupiter","Saturn"}; ArrayAdapter<String> adapter = new ArrayAdapter<String>(this, android.R.layout.simple_spinner_item, this.arrayPlanet); Spinner s = (Spinner) findViewById(R.id.spinnerPlanet); s.setOnItemSelectedListener(new OnItemSelectedListener() { @Override public void onItemSelected(AdapterView<?> arg0, View arg1, int position, long id) { if (position > 0) { selectPlanet = String.valueOf(position - 1); } else { selectPlanet = null; } } @Override public void onNothingSelected(AdapterView<?> arg0) { } }); s.setAdapter(adapter); new Thread(new ClientThread()).start(); } @Override protected void onDestroy() { if (!socket.isClosed()) { try { DataOutputStream output = new DataOutputStream(socket.getOutputStream()); output.writeUTF("exit"); } catch (UnknownHostException e) { e.printStackTrace(); } catch (IOException e) { e.printStackTrace(); } catch (Exception e) { e.printStackTrace(); } } super.onDestroy(); } public void onClickLocalCalculate(View view) { EditText et = (EditText) findViewById(R.id.editTextAge); String result; if (selectPlanet == null) { StringBuilder strBuilder = new StringBuilder(""); for (int i = 0; i < 4; i++) { strBuilder.append(calculateAgeOnPlanet(Double.valueOf(et.getText().toString()), i) + "\r\n"); } result = String.format("local: %s", strBuilder.toString()); } else { result = String.format("local: %s", calculateAgeOnPlanet(Double.valueOf(et.getText().toString()), Integer.valueOf(selectPlanet))); } TextView txt = (TextView) findViewById(R.id.textViewAge); txt.setText(result); } private String calculateAgeOnPlanet(double ageInEarth, int planetIndex) { String[] namePlanet = new String[] {"Mercury","Venus","Jupiter","Saturn"}; double[] dayPlanet = new double[] {87.96, 224.68, 11.862, 29.456}; return "Age " + ageInEarth + " in earth = age " + getAgeOnPlanet(ageInEarth, dayPlanet[planetIndex]) + " in " + namePlanet[planetIndex]; } private double getAgeOnPlanet(double ageInEarth, double dayPlanetBaseEarth) { return ageInEarth * 365 / dayPlanetBaseEarth; } public void onClickSendCalculate(View view) { if (socket.isClosed()) Toast.makeText(this.getBaseContext(), "Socket close restart aplikasi...", Toast.LENGTH_SHORT).show(); try { EditText et = (EditText) findViewById(R.id.editTextAge); String str; if (selectPlanet == null) { str = "#calcAge" + ":" + et.getText().toString(); } else { str = "#calcAge" + ":" + et.getText().toString() + ":" + selectPlanet; } DataOutputStream output = new DataOutputStream(socket.getOutputStream()); output.writeUTF(str); new ReceiveMessageFromServer().execute(""); } catch (UnknownHostException e) { e.printStackTrace(); } catch (IOException e) { e.printStackTrace(); } catch (Exception e) { e.printStackTrace(); } } class ReceiveMessageFromServer extends AsyncTask<String, Void, String> { @Override protected String doInBackground(String... params) { DataInputStream input; String response = ""; try { input = new DataInputStream(socket.getInputStream()); response = input.readUTF(); } catch (IOException e) { e.printStackTrace(); } return response; } @Override protected void onPostExecute(String result) { TextView txt = (TextView) findViewById(R.id.textViewAge); txt.setText(result); if (result.equalsIgnoreCase("bye")) { try { socket.close(); } catch (IOException e) { e.printStackTrace(); } } } @Override protected void onPreExecute() { } @Override protected void onProgressUpdate(Void... values) { } } class ClientThread implements Runnable { @Override public void run() { try { InetAddress serverAddr = InetAddress.getByName(SERVER_IP); socket = new Socket(serverAddr, SERVERPORT); } catch (UnknownHostException e1) { e1.printStackTrace(); } catch (IOException e1) { e1.printStackTrace(); } } } } |
file “activity_age_planet.xml” yang berada di res/layout:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 |
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="fill_parent" android:layout_height="fill_parent" android:orientation="vertical" > <ScrollView android:layout_width="fill_parent" android:layout_height="wrap_content"> <LinearLayout android:layout_width="fill_parent" android:layout_height="wrap_content" android:orientation="vertical"> <EditText android:id="@+id/editTextAge" android:layout_width="fill_parent" android:layout_height="wrap_content" android:inputType="number" android:text="" > </EditText> <Spinner android:id="@+id/spinnerPlanet" android:layout_width="match_parent" android:layout_height="wrap_content" /> <Button android:id="@+id/buttonLocalCalculateAge" android:layout_width="match_parent" android:layout_height="wrap_content" android:onClick="onClickLocalCalculate" android:text="Calculate" > </Button> <Button android:id="@+id/buttonSendCalculateAge" android:layout_width="match_parent" android:layout_height="wrap_content" android:onClick="onClickSendCalculate" android:text="Send to Calculate" /> <TextView android:id="@+id/textViewAge" android:layout_width="match_parent" android:layout_height="wrap_content" android:text="" /> </LinearLayout> </ScrollView> </LinearLayout> |
Rubah file “AndroidManifest.xml” menjadi:
1 2 3 4 5 6 7 8 9 |
<activity android:name=".AgePlanetActivity" android:label="@string/title_activity_age_planet" > <intent-filter> <action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.LAUNCHER" /> </intent-filter> </activity> |
Mantaappp om…sukses slalu #Up
Amiin, thanks om.